There are times when we would like our threads to start at the same time or wait for some operation that another thread is performing. Alternatively, we might want to record the timestamp when the last thread finished execution in a multi-threaded process.
If we use the join method in the java.lang.Thread class, then each thread has to complete its execution and only then can the next thread start execution. Let’s say we have 10 threads. Once Thread 0 kicks in , Threads 1-9 have to keep waiting on Thread-0, then lets say Thread 1 kicks in(post completion of Thread 0), then Threads 2-9 keep waiting. So, the join method is never gonna be of great help in this case. Take a look at the example code below:
import java.lang.*;
public class ThreadJoin implements Runnable {
public void run() {
Thread t = Thread.currentThread(); System.out.println(“Current thread : ” + t.getName());
}
public static void main(String args[]) throws Exception {
for (int i = 0; i < 10; i++) { Thread t = new Thread(new ThreadJoin()); // this will call run() function t.start(); // waits for this thread to die,and only then can you go to the next thread t.join(); System.out.println(t.getName() + ” has ended”+”\n——————–\n”); }
System.out.println(“Finally at the end of all the threads!!!”); }
}
This is what you get when you run the above code.
We can clearly see that each thread is waiting on its predecessor to complete its job and only then does it have a chance to get in.
So, how do we let them all run in parallel but still wait on each one of them to finish their job, before exiting the application.
The java.util.concurrent package has the CountDownLatch class for this purpose.
The CountDownLatch is:
a synchronization aid that allows one or more threads to wait until a set of operations being performed in other threads completes.
So how does this work?
The class provides two methods for this purpose :
countDown() and await()
Our requirement here is to wait till all the threads finish execution.
Lets get down to do some serious coding now!
We shall have 10 threads that shall be running in parallel. So, we shall initialize the CountDownLatch to 10.
Each thread shall then call the countDown() method, that would count the latch down or let’s say decrement it, by 1 once it completes execution. Once the last thread would do the same, the latch would be set to zero. Meanwhile the other threads would realize that their super-slow last buddy has also finished execution and they would be all happy to perform a collective suicide.
Lets use this example code to understand how it all happens:
import java.util.concurrent.CountDownLatch;
class StopLatchedThread extends Thread {
private final CountDownLatch stopLatch;
public StopLatchedThread(CountDownLatch stopLatch) { this.stopLatch = stopLatch; } public void run() { Thread t = Thread.currentThread(); System.out.println(“Current thread : ” + t.getName()); try { // perform some task here } finally { stopLatch.countDown();//once a thread completes execution, it counts the latch down (say , count–) System.out.println(“\nThread : ” + t.getName() + ” has brought the latch down to ” + stopLatch.getCount()+”\n”); } } }
public class ThreadCountDownLatch{ public static void main(String []args) throws InterruptedException { CountDownLatch cdl = new CountDownLatch(10); for (int i = 0; i < 10; i++) { Thread t = new StopLatchedThread(cdl); System.out.println(t.getName() + ” has been started”); t.start(); } cdl.await(); // this is where all the threads are waiting for their last buddy to finish execution System.out.println(“\nCongratulations. Finally all the threads have done their jobs. Now lets die together!!!”); }
}
Run the code above and you get this:
We can see here that the threads started to run in parallel mode. Then once the last one(which was Thread-9 in my case) counted the latch down to ‘0’, all the other threads who were “awaiting” this event, were notified and they all died together(along with Thread-9).
Where can one employ CountDownLatch?
Like we did in the example above, one might need to know when a multi-thread application stops or when the last thread completes execution(of course, there are other ways to do this). Instead of putting a counter for each thread and then taking the end time of the last one, we can just use this aid.
One might want to test the load his/her website can take. It would be a good choice to use the CountDownLatch so that we can start hundred or thousands at more-or-less the same time.
There are times when we would like our threads to start at the same time or wait for some operation that another thread is performing. Alternatively, we might want to record the timestamp when the last thread finished execution in a multi-threaded process.
If we use the join method in the java.lang.Thread class, then each thread has to complete its execution and only then can the next thread start execution. Let’s say we have 10 threads. Once Thread 0 kicks in , Threads 1-9 have to keep waiting on Thread-0, then lets say Thread 1 kicks in(post completion of Thread 0), then Threads 2-9 keep waiting. So, the join method is never gonna be of great help in this case. Take a look at the example code below:
This is what you get when you run the above code.
We can clearly see that each thread is waiting on its predecessor to complete its job and only then does it have a chance to get in.
So, how do we let them all run in parallel but still wait on each one of them to finish their job, before exiting the application.
The java.util.concurrent package has the CountDownLatch class for this purpose.
The CountDownLatch is:
So how does this work?
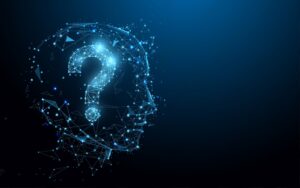
The class provides two methods for this purpose :
Our requirement here is to wait till all the threads finish execution.
Lets get down to do some serious coding now!
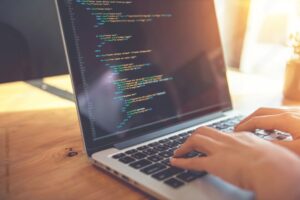
We shall have 10 threads that shall be running in parallel. So, we shall initialize the CountDownLatch to 10.
Each thread shall then call the countDown() method, that would count the latch down or let’s say decrement it, by 1 once it completes execution. Once the last thread would do the same, the latch would be set to zero.
Meanwhile the other threads would realize that their super-slow last buddy has also finished execution and they would be all happy to perform a collective suicide.
Lets use this example code to understand how it all happens:
Run the code above and you get this:
We can see here that the threads started to run in parallel mode. Then once the last one(which was Thread-9 in my case) counted the latch down to ‘0’, all the other threads who were “awaiting” this event, were notified and they all died together(along with Thread-9).
Where can one employ CountDownLatch?
Recent Posts
Cost Saving: $25,000* Year-over-Year for Our Client
October 3, 2023Comparative Analysis of Integration Engine
October 3, 2023Selecting the Perfect Interface Engine for Your
September 14, 2023Synchronization And The Atomic Integer
August 14, 2023ERP Solutions for Contract Lifecycle Management
August 1, 2023Recent Comments
Popular Categories
Archives